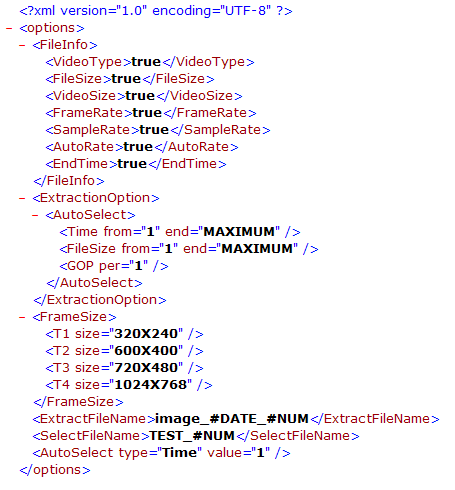
import java.io.*;
import org.jdom.*;
import org.jdom.output.*;
/**
* JDom을 이용한 파서 예제
* @author won
*
*/
public class MakeXmlWithJDom {
public static void main(String [] args) {
new MakeXmlWithJDom();
}
public MakeXmlWithJDom(){
/*
* Create Root Element
*/
Document doc = new Document();
Element rootElement = new Element("options");
doc.addContent(rootElement);
/*
* Add FileInfo Element
*/
String[] inpFileElements = {"VideoType","FileSize","VideoSize","FrameRate","SampleRate","AutoRate","EndTime"};
String[] inpFileValue = {"true","true","true","true","true","true","true"};
Element fileInfoElement = new Element("FileInfo");
for(int i=0;i<inpFileElements.length;i++){
addInnerElement(fileInfoElement, inpFileElements[i],inpFileValue[i]);
}
rootElement.addContent(fileInfoElement);
/*
* Add ExtractionOption Element
*/
String inpAutoExtracValue = "true";
String[] inpAutoElement = {"Time","FileSize","GOP"};
String[][] inpAutoAttName = {{"from","end"},{"from","end"},{"per"}};
String[][] inpAutoAttValue = {{"1","MAXIMUM"},{"1","MAXIMUM"},{"1"}};
Element extractionOption = new Element("ExtractionOption");
Element elAutoSelect = addInnerElement(extractionOption, "AutoSelect", "");
for(int i=0;i<inpAutoElement.length;i++){
Element tmpElement = addInnerElement(elAutoSelect, inpAutoElement[i],"");
for(int j=0;j<inpAutoAttName[i].length;j++){
addInnerAttribute(tmpElement, inpAutoAttName[i][j], inpAutoAttValue[i][j]);
}
}
rootElement.addContent(extractionOption);
/*
* Add FrameSize Element
*/
String[] inpFrameSize = {"320X240","600X400","720X480","1024X768"};
Element frameSize = new Element("FrameSize");
for(int i=0;i<inpFrameSize.length;i++){
Element tmpElement = addInnerElement(frameSize, "T"+(i+1),"");
addInnerAttribute(tmpElement, "size", inpFrameSize[i]);
}
rootElement.addContent(frameSize);
/*
* Add ExtractFileName Element
*/
String inpExtractFileName="image_#DATE_#NUM";
Element extractFileName = new Element("ExtractFileName");
extractFileName.setText(inpExtractFileName);
rootElement.addContent(extractFileName);
/*
* Add SelectFileName Element
*/
String inpSelectFileName="TEST_#NUM";
Element selectFileName = new Element("SelectFileName");
selectFileName.setText(inpSelectFileName);
rootElement.addContent(selectFileName);
/*
* Add AutoSelect Element
*/
String inpAutoType="Time";
String inpAutoValue="1";
Element autoSelect = new Element("AutoSelect");
addInnerAttribute(autoSelect,"type",inpAutoType);
addInnerAttribute(autoSelect,"value",inpAutoValue);
rootElement.addContent(autoSelect);
/*
* Preparing for printout
*/
XMLOutputter outp = new XMLOutputter();
/*
* file output
*/
try {
OutputStream fos = new FileOutputStream("C:\\mmm.xml");
outp.output(doc, fos);
} catch (Exception e) {
e.printStackTrace();
}
/*
* screen output
*/
/*
try{
outp.output(doc, System.out);
}
catch (IOException e) {
System.out.println(e);
}
*/
}
/**
* 엘레멘트 하위 요소를 추가하여 준다.
* @param target 추가하려는 엘레멘트
* @param eElement 신규 엘레멘트 이름
* @param eValue 신규 엘레멘트 값
* @return 신규 엘레멘트
*/
public Element addInnerElement(Element target, String eElement, String eValue){
Element newElement = new Element(eElement);
newElement.setText(eValue);
target.addContent(newElement);
return newElement;
}
/**
* <pre>
* 엘레먼트 내부에 어트리뷰트를 추가하여 준다.
* 추가시 입력된 순서대로 추가된다.
* </pre>
* @param target 추가하려는 엘레먼트
* @param attName 어트리뷰트 이름
* @param attValue 어트리뷰트 값
*/
public void addInnerAttribute(Element target, String attName, String attValue){
Attribute newElement = new Attribute(attName,attValue);
target.setAttribute(newElement);
}
};
사람 눈에 맞춰 문서의 인쇄 상태를 보기 좋게 만들려면 포멧을 변경하면 된다.
Format fm = Format.getPrettyFormat();
XMLOutputter outp = new XMLOutputter(fm);
자세한 내용은 org.jdom.output.Format 를 참조하여 변경하면 된다.
참조 사이트 : http://www.javaworld.com/javaworld/jw-05-2000/jw-0518-jdom.html
'etc > old' 카테고리의 다른 글
2008년 4월 신작 자막 제작자 정리표 (0) | 2008.06.03 |
---|---|
InputStream, OutputStream 쓰자.. (파일 읽고 쓰기) (0) | 2008.06.02 |
JDom 관련 문서 (0) | 2008.06.02 |
JDOM으로 XML 프로그래밍 단순화 하기 (한글) (0) | 2008.06.02 |
Returning a JDBC result set from an Oracle stored procedure (0) | 2008.05.30 |